Guide: How to Install Dependencies from Package.json Using Yarn
If you’re a developer working on a project, you know how important it is to manage and install dependencies. Dependencies are essential components that your project relies on to function correctly. In this guide, we’ll show you how to use Yarn to install dependencies from a package.json file, a standard method used in modern web development. With Yarn, you can easily manage your project’s dependencies, ensuring all components are up-to-date and functioning correctly.
Master installing dependencies from package.json using Yarn, and learn the essential steps to add dev dependencies effectively.
Key Takeaways:
- Yarn is a powerful tool for managing dependencies in your project.
- A package.json file stores information about your project and its dependencies.
- With Yarn, you can easily install, manage, and remove dependencies from your project.
- Yarn’s dependency resolution system ensures that all installed components are compatible.
Understanding Package.json and Dependencies
Every Node.js project requires specific dependencies to function correctly. The package.json file in your project’s root directory lists all the dependencies your project requires.
To ensure a successful installation of all required dependencies, it is crucial to understand the package.json file and its dependencies. Yarn is a reliable and efficient package manager that simplifies managing dependencies in your project.
What is Package.json?
Package.json is a file that lists the metadata and dependencies of a Node.js project. It helps in managing the installation of project dependencies with Yarn.
The file contains information about the name, version, author, and project description. It also specifies the required dependencies of the project and their versions. Whenever a dependency is added to or removed from a project, the package.json file should be updated accordingly.
What are Dependencies?
Dependencies are external modules that a Node.js project requires to work correctly. They can include libraries, frameworks, and other software packages. The dependencies listed in the package.json file tell Yarn which packages to download and install when a project is set up on a new machine or after a fresh installation.
These dependencies are necessary for the project to run correctly. This is why ensuring that all dependencies are listed in the package.json file and correctly installed with Yarn is essential.
Installing Yarn and Setting Up the Project
Before installing dependencies with Yarn, you must install Yarn on your system. To install Yarn, follow the instructions on the official Yarn website.
Once Yarn is installed, navigate to your project directory in the terminal. If you don’t have a project directory yet, create one using the following command:
mkdir project-name
Next, navigate to the project directory:
cd project-name
Now, you can initialize a package.json file by running the following command:
yarn init
This will lead you to respond to inquiries regarding your project, including details like the project’s name and version number. Once you have answered all the questions, Yarn will generate a package.json file in your project directory.
Now that you have a package.json file, you can install dependencies with Yarn.
Understanding Yarn’s Dependency Resolution
Yarn’s dependency resolution is crucial to managing dependencies in a project. When installing dependencies from a package.json file, Yarn looks at each dependency’s version requirements and determines the best possible combination of versions that satisfies all dependencies. This process is known as dependency resolution.
Yarn uses a versioning system called semantic versioning, also known as SemVer, to define dependency versions. SemVer consists of three parts: the major version, the minor version, and the patch version. When defining dependencies in a package.json file, you can specify a range of acceptable versions using different operators, such as ^, ~, =, and more.
Yarn checks the package.json file’s dependencies during installation to determine which packages to download and install. Yarn also creates a yarn.lock file that specifies the exact version of each installed package and its dependencies. This file ensures all team members use the identical package versions, avoiding conflicts and compatibility issues.
Version Compatibility and Resolving Conflicts
One of the benefits of using Yarn is that it helps resolve conflicts between different package versions. If two or more dependencies have conflicting version requirements, Yarn will install the version that best satisfies all other dependencies. Sometimes, Yarn may ask you to manually resolve a conflict by selecting the version you want to install.
It’s important to note that sometimes, upgrading a package to a newer version may cause compatibility issues with other dependencies. Yarn allows you to specify an exact version of a package to ensure that it works with the rest of the project’s dependencies. If necessary, you can also roll back to a previous package version.
Conclusion
Understanding how Yarn’s dependency resolution works is essential for efficient package management. By using a combination of semantic versioning, dependency resolution, and the Yarn.lock file, Yarn ensures that packages are installed consistently across different environments and team members.
Installing Dependencies with Yarn
Now that we have set up our project with Yarn, it’s time to install the required dependencies. Installing dependencies with Yarn is a straightforward process that can be completed with the simple command yarn install. This instruction will initiate downloading all required packages and their dependencies, if any, from the package.json file and subsequently install them within the project.
If your package.json file has any dependencies already installed in the project, running yarn install will update them to the latest version specified in the file. Additionally, if you want to install a specific package version, specify it in the Yarn add command, along with the package name and version number.
It’s important to note that you can also use the yarn install command to install dependencies from other sources besides the package.json file. For example, you can install a package directly from the internet by specifying its URL.
Yarn also provides several options that can be used with the yarn install command. For example, the —-production flag can install only the production-level dependencies and exclude development dependencies.
Another useful option is –ignore-engines, which allows you to bypass the compatibility check for package engines and install the dependencies regardless of potential conflicts.
Step-by-Step Guide to Installing Dependencies with Yarn
Here is a step-by-step guide to installing dependencies with Yarn:
- Go to the project folder within your terminal by using the navigation commands.
- Run the command yarn install.
- Wait for Yarn to download and install all the necessary packages and dependencies.
- Verify that the packages have been installed by checking the node_modules directory.
It’s that simple! With Yarn, you can install all your project dependencies with just a few keystrokes.
Managing Package Versions with Yarn
When working with dependencies in a project, managing package versions is essential. Yarn offers several options for installing and managing package versions from a package.json file.
One of the most valuable features of Yarn is its ability to install specific versions of packages. This can be done using the Yarn add [package-name]@[version-number] command. For example, if you need to install version 2.2.1 of the react package, you can run the following command:
yarn add react@2.2.1
You can also update package versions in the package.json file using the yarn upgrade [package-name] command. This command will update the package to the latest version within the defined version range specified in the package.json file. For example, if the package.json file specifies a version range of “^2.0.0” for the react package, running the following command will update it to the latest version within that range:
Yarn upgrade react
It is important to note that updating a package version may cause compatibility issues with other packages in the project. To avoid this, testing the updated version thoroughly before deploying it to production is recommended.
When working with dependencies, it is also essential to understand Yarn’s versioning system. Yarn uses semantic versioning, which consists of three numbers separated by periods (e.g., 1.2.3). The first number represents significant changes that are not backward-compatible, the second number represents minor changes backward-compatible, and the third number represents patches or bug fixes.
Finally, updating package versions in the package.json file can be done manually by changing the version number in the file, or it can be done automatically using the yarn version [version-number] command. By running this command, you will update the version number in the package.json file and generate a new Git tag to represent that version.
In conclusion, managing package versions is essential to working with dependencies in a project. Yarn provides several options for installing and managing package versions from a package.json file, including installing specific versions, updating to the latest version within a specified range, and manually or automatically updating the version number in the package.json file. By understanding Yarn’s versioning system and using its version management features, developers can ensure their projects are up-to-date and compatible with all required dependencies.
Removing Dependencies with Yarn
At times, removing specific dependencies from a project may be necessary. This could be due to compatibility issues, conflicts with other packages, or simply because they’re no longer needed. Using the Yarn Remove command, Yarn provides an easy way to remove dependencies from a project.
To eliminate a particular package from a project, proceed to the project directory using your terminal and then execute the following command:
Yarn remove package-name
Substitute “package-name” with the actual name of the package you wish to remove. Yarn will remove the package and update the package.json file accordingly, removing the package from the dependencies list.
If you want to remove all dependencies from a project altogether, you can delete the node_modules directory in the project’s root folder and then execute the following command:
yarn
This will reinstall all dependencies listed in the package.json file.
It’s important to note that removing a dependency may have unintended consequences, such as breaking the functionality of other packages that depend on it. Use caution when removing dependencies, and test your project thoroughly after making any changes.
Resolving Common Issues with Dependency Installation
Installing dependencies from a package.json file using Yarn is a straightforward process. However, there may be times when you encounter issues during installation. Here are some common issues you might encounter and how to resolve them:
1. Conflicts between dependencies
Conflicts between dependencies can occur when two packages require different versions of the same package or when two packages have incompatible dependencies. One solution is to use Yarn’s resolutions field in the package.json file to specify the package version that should be used.
For example, to force the use of version 2.5.0 of the package “example-package” instead of version 3.0.0:
“resolutions”: {
“example-package”: “2.5.0”
}
2. Missing dependencies
If Yarn cannot find a dependency during installation, it could be because the package is unavailable in the registry or misspelled. Please double-check the package name’s spelling and ensure it is available in the registry.
If the package is not available in the registry, you can try adding a custom registry to your .npmrc file:
registry=https://custom-registry.com
3. Compatibility issues
Compatibility issues can arise when a package is incompatible with the version of Node.js or your operating system. One solution is to use Yarn’s –ignore-engines flag during installation to skip dependency compatibility checks.
For example:
Yarn install –ignore-engines
However, skipping compatibility checks may result in unexpected behavior or errors in your project.
These are just a few common issues you may encounter when installing dependencies with Yarn. If you encounter any other issues, consult the Yarn documentation or seek help from the community.
Automating Dependency Installation with Yarn Scripts
As projects grow in complexity, dependency installation can become a time-consuming task. Thankfully, Yarn solves this problem by allowing you to automate your dependency installation process using Yarn scripts. This section will guide you through setting up and using Yarn scripts to install package.json dependencies.
Defining Yarn Scripts in package.json
Before using Yarn scripts, you must define them in the package.json file. To do so, add a new entry under the “scripts” field with your chosen name and a command corresponding to the script you want to execute. For example:
“scripts”: {
“setup”: “yarn install,”
“start”: “node index.js”
}
In this example, we have defined two Yarn scripts: “setup” and “start”. The “setup” script runs the command “yarn install” to install all dependencies listed in the package.json file. The “start” script runs the “node index.js” command to start the project.
Running Yarn Scripts
To run a Yarn script, use the command “yarn run” followed by the script name. For example, to run the “setup” script defined in the previous example, enter:
Yarn run setup
This will execute the “yarn install” command and install all dependencies listed in the package.json file.
Benefits of Using Yarn Scripts
Using Yarn scripts to automate dependency installation has several benefits:
- Efficient: Yarn scripts allow you to execute multiple commands with a single command, saving you time and effort.
- Customizable: You can define custom scripts to suit your project’s needs and simplify your workflow.
- Consistent: Yarn scripts ensure that everyone working on the project uses the same installation and setup processes.
By implementing Yarn scripts in your workflow, you can streamline your dependency installation process, making it more efficient and customizable.
Conclusion
Installing dependencies is crucial for any project’s functionality, and Yarn makes the process easy and efficient. By understanding the ins and outs of package.json and its dependencies, you can use Yarn’s many features to streamline your workflow and keep your project up-to-date.
Using Yarn to install package.json dependencies is straightforward, with the yarn install command allowing you to manage your packages easily. Yarn’s dependency resolution system ensures version compatibility and resolves conflicts, making it a reliable choice for project management.
When issues arise, Yarn provides troubleshooting tips and solutions to keep your project running smoothly. And, if you want to automate your dependency installation process, custom scripts in the package.json file can do the work for you.
The Benefits of Yarn
Yarn offers many advantages over other dependency managers, including faster installation times and improved performance. Its caching system lets you install packages quickly, and its lock file ensures consistent installations across different systems.
Overall, Yarn is a powerful tool for managing project dependencies, and installing package.json dependencies with Yarn is a skill worth mastering. Start implementing this knowledge in your projects to streamline workflow and maximize efficiency.
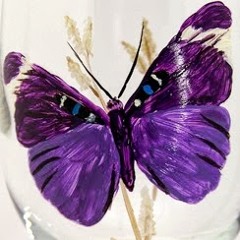
Vera Mondragon, a luminary in the yarn industry, weaves together her passion and expertise. With a knack for creativity and a love for all things yarn, Vera guides enthusiasts through the vibrant world of fibers, offering insights and inspiration for every crafting journey.